Introduction
PrimeStego is an innovative application that combines the use of Amazon Prime with steganography to offer a secure and convenient solution for sharing sensitive data. With PrimeStego, you can easily hide ZIP files containing your confidential data within dynamically generated PNG images. These images can then be uploaded to Amazon Prime Photos, which offers unlimited storage for Prime members.
How it Works
PrimeStego utilizes steganography, the art of concealing information within other non-secret data, to embed ZIP files into PNG images. The process involves generating PNG images with random shapes and text, then hiding the ZIP files within these images. The resulting images appear normal to the naked eye but contain hidden data that can be extracted using PrimeStego.
Features
- Secure Data Sharing: PrimeStego offers a secure way to share sensitive data by concealing it within images.
- Amazon Prime Integration: Leveraging the unlimited storage offered by Amazon Prime Photos, PrimeStego ensures that your hidden data is safely stored in the cloud.
- Dynamic Image Generation: Images are dynamically generated with random shapes, text, and colors, making each image unique and difficult to detect.
- Easy Extraction: Extracting hidden ZIP files from images is simple and straightforward with PrimeStego.
Usage
To use PrimeStego:
- Generate JPG images with hidden ZIP files using the PrimeStego application.
- Upload these images to Amazon Prime Photos.
- Share the images with intended recipients.
- Recipients can then use PrimeStego to extract the hidden ZIP files from the images.
Installation
- Clone the PrimeStego repository to your local machine.
- Run the PrimeStego application.
- Follow the on-screen instructions to generate images with hidden ZIP files.
Create.ps1
# Définir les dossiers
$zipFolder = "C:\MyData\zip"
$outputFolder = "C:\MyData\photos"
# Créer le dossier de sortie s'il n'existe pas
if (-Not (Test-Path $outputFolder)) {
New-Item -ItemType Directory -Path $outputFolder
}
# Fonction pour obtenir la taille du fichier en Mo
function Get-FileSizeInMB {
param (
[string]$filePath
)
$fileInfo = Get-Item $filePath
return [math]::Round($fileInfo.Length / 1MB, 2)
}
# Fonction pour générer une image avec des formes et des couleurs aléatoires
function Generate-RandomImage {
param (
[string]$outputFilePath,
[string]$fileName,
[int]$width = 3840,
[int]$height = 2160,
[int]$maxShapes = 10
)
Add-Type -AssemblyName System.Drawing
$bitmap = New-Object System.Drawing.Bitmap $width, $height
$graphics = [System.Drawing.Graphics]::FromImage($bitmap)
$graphics.Clear([System.Drawing.Color]::Black)
$random = New-Object System.Random
$brushes = [System.Collections.ArrayList]@()
# Ajouter des couleurs aléatoires aux pinceaux
1..$maxShapes | ForEach-Object {
$brushes.Add([System.Drawing.SolidBrush]::new([System.Drawing.Color]::FromArgb(
$random.Next(0, 256), # R
$random.Next(0, 256), # G
$random.Next(0, 256) # B
)))
}
# Dessiner des formes aléatoires
1..$random.Next(1, $maxShapes) | ForEach-Object {
$shapeType = $random.Next(0, 5) # 0: rectangle, 1: cercle, 2: triangle, 3: trait, 4: pixel
$colorIndex = $random.Next(0, $brushes.Count)
switch ($shapeType) {
0 { # Rectangle
$x = $random.Next(0, $width - 200)
$y = $random.Next(0, $height - 200)
$rectangle = [System.Drawing.Rectangle]::new($x, $y, 200, 200)
$graphics.FillRectangle($brushes[$colorIndex], $rectangle)
}
1 { # Cercle
$x = $random.Next(0, $width - 200)
$y = $random.Next(0, $height - 200)
$rectangle = [System.Drawing.Rectangle]::new($x, $y, 200, 200)
$graphics.FillEllipse($brushes[$colorIndex], $rectangle)
}
2 { # Triangle
$points = @(
[System.Drawing.Point]::new($random.Next(0, $width), $random.Next(0, $height)),
[System.Drawing.Point]::new($random.Next(0, $width), $random.Next(0, $height)),
[System.Drawing.Point]::new($random.Next(0, $width), $random.Next(0, $height))
)
$graphics.FillPolygon($brushes[$colorIndex], $points)
}
3 { # Trait
$x1 = $random.Next(0, $width)
$y1 = $random.Next(0, $height)
$x2 = $random.Next(0, $width)
$y2 = $random.Next(0, $height)
$graphics.DrawLine([System.Drawing.Pen]::new($brushes[$colorIndex].Color), $x1, $y1, $x2, $y2)
}
4 { # Pixel
$x = $random.Next(0, $width)
$y = $random.Next(0, $height)
$graphics.FillRectangle($brushes[$colorIndex], $x, $y, 1, 1)
}
}
}
# Afficher le nom du fichier sans l'extension .zip à un endroit aléatoire
$fontSize = $random.Next(24, 60)
$font = [System.Drawing.Font]::new("Arial", $fontSize)
# Calculer la taille du texte pour s'assurer qu'il soit entièrement visible
$textSize = $graphics.MeasureString($fileName, $font)
$textX = $random.Next(0, [math]::Max(0, $width - $textSize.Width))
$textY = $random.Next(0, [math]::Max(0, $height - $textSize.Height))
$graphics.DrawString($fileName, $font, [System.Drawing.SolidBrush]::new([System.Drawing.Color]::White), $textX, $textY)
$bitmap.Save($outputFilePath, [System.Drawing.Imaging.ImageFormat]::Jpeg)
$bitmap.Dispose()
$graphics.Dispose()
}
# Fonction pour cacher un fichier ZIP dans une image
function Hide-ZipInImage {
param (
[string]$zipFilePath,
[string]$imageFilePath,
[string]$outputFilePath
)
# Lire le contenu de l'image et du fichier ZIP
$imageBytes = [System.IO.File]::ReadAllBytes($imageFilePath)
$zipBytes = [System.IO.File]::ReadAllBytes($zipFilePath)
# Combiner les octets de l'image et du fichier ZIP
$combinedBytes = $imageBytes + $zipBytes
# Écrire les octets combinés dans le fichier de sortie
[System.IO.File]::WriteAllBytes($outputFilePath, $combinedBytes)
}
# Parcourir les fichiers ZIP dans le dossier
Get-ChildItem -Path $zipFolder -Filter *.zip | ForEach-Object {
$zipFile = $_
# Générer une image avec des formes et des couleurs aléatoires
$imageFileName = "$($zipFile.BaseName).jpg"
$imageFilePath = Join-Path -Path $outputFolder -ChildPath $imageFileName
Generate-RandomImage -outputFilePath $imageFilePath -fileName $zipFile.BaseName
# Créer le chemin de sortie pour l'image stéganographique avec le nom du fichier ZIP
$outputFilePath = Join-Path -Path $outputFolder -ChildPath $imageFileName
# Cacher le fichier ZIP dans l'image
Hide-ZipInImage -zipFilePath $zipFile.FullName -imageFilePath $imageFilePath -outputFilePath $outputFilePath
}
Extract.ps1
# Définir le dossier contenant les images stéganographiques et le dossier de sortie
$inputFolder = "C:\MyData\photos"
$outputFolder = "C:\MyData\zip_extracted"
# Créer le dossier de sortie s'il n'existe pas
if (-Not (Test-Path $outputFolder)) {
New-Item -ItemType Directory -Path $outputFolder
}
# Fonction pour extraire le fichier ZIP de l'image stéganographique
function Extract-ZipFromImage {
param (
[string]$imageFilePath,
[string]$outputFolderPath
)
$imageBytes = [System.IO.File]::ReadAllBytes($imageFilePath)
# Définition des en-têtes de fichier ZIP
$zipHeader = [byte[]](0x50, 0x4B, 0x03, 0x04) # En-tête du fichier ZIP en bytes
# Recherche de l'index du premier en-tête de fichier ZIP
$zipIndex = 0
for ($i = 0; $i -lt ($imageBytes.Length - 3); $i++) {
$match = $true
for ($j = 0; $j -lt 4; $j++) {
if ($imageBytes[$i + $j] -ne $zipHeader[$j]) {
$match = $false
break
}
}
if ($match) {
$zipIndex = $i
break
}
}
if ($zipIndex -ne 0) {
# Extraire le contenu du fichier ZIP à partir de l'index trouvé
$zipContent = $imageBytes[$zipIndex..($imageBytes.Length - 1)]
$outputFilePath = Join-Path -Path $outputFolderPath -ChildPath ([System.IO.Path]::GetFileNameWithoutExtension($imageFilePath) + ".zip")
[System.IO.File]::WriteAllBytes($outputFilePath, $zipContent)
Write-Host "Fichier ZIP extrait avec succès : $outputFilePath"
} else {
Write-Host "Aucun fichier ZIP trouvé dans l'image : $imageFilePath"
}
}
# Parcourir les images stéganographiques dans le dossier
Get-ChildItem -Path $inputFolder -Filter *.jpg | ForEach-Object {
$imageFile = $_
Extract-ZipFromImage -imageFilePath $imageFile.FullName -outputFolderPath $outputFolder
}
Git >> https://github.com/mike37510/PrimeStego/
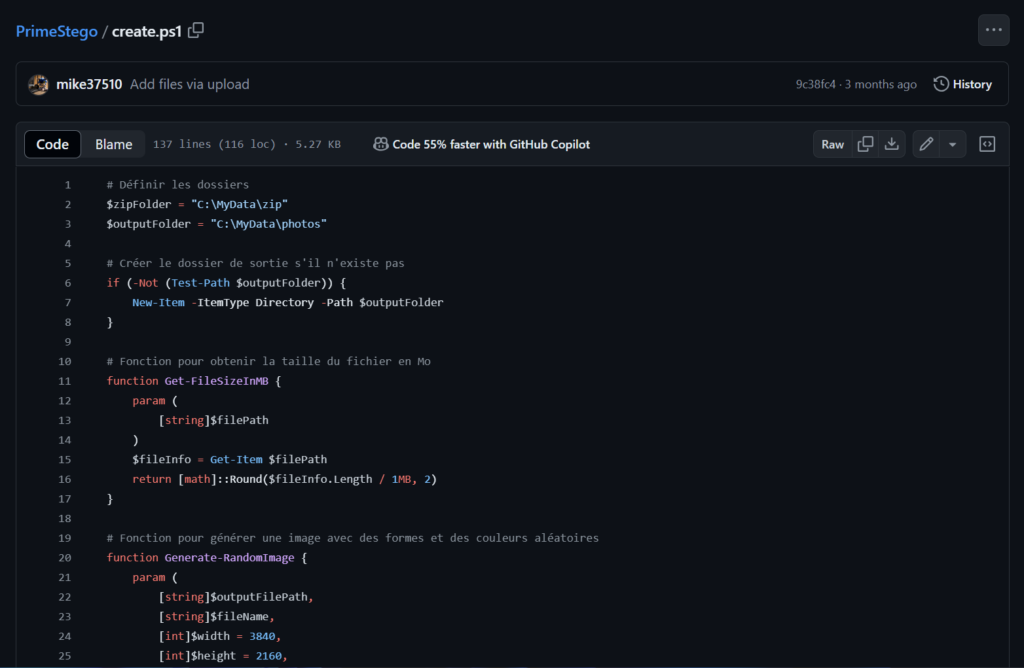